Reactive Twig Templates
An Interactive UI
in PHP & Twig
Develop your interface.. from the comfort of Symfony.
No Javascript needed.
composer require symfony/ux-live-component
// ... use statements hidden - click to show
#[AsLiveComponent('SearchPackages', template: 'components/Package/SearchPackages.html.twig')]
class SearchPackages
{
use DefaultActionTrait;
#[LiveProp(writable: true, url: true)]
public ?string $query = null;
public function __construct(private UxPackageRepository $packageRepo)
{
}
public function getPackages(): array
{
return $this->packageRepo->findAll($this->query);
}
}
<div{{ attributes }}>
<input type="text" data-model="query" placeholder="Results update as you type..." class="form-control" />
<div class="PackageList pt-3" data-loading="addClass(opacity-50)">
{% for package in computed.packages %}
{{ include('components/Package/PackageListItem.html.twig', {package}) }}
{% else %}
No packages found "{{ query }}"
{% endfor %}
</div>
</div>

Live Components
Install It
$ composer require symfony/ux-live-component
Live Component Demos
Find out what else you can build
Read full Documentation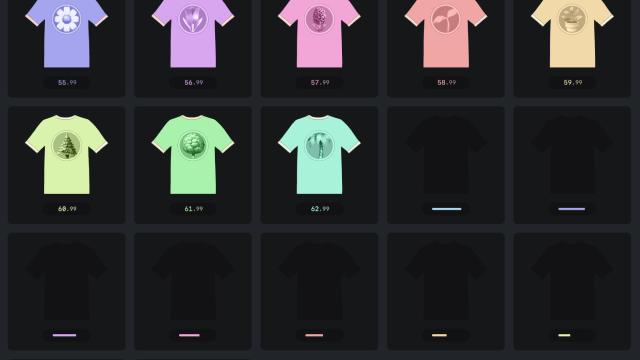
Infinite Scroll - 2/2
Loading on-scroll, flexible layout grid, colorfull loading animations and... more T-Shirts!
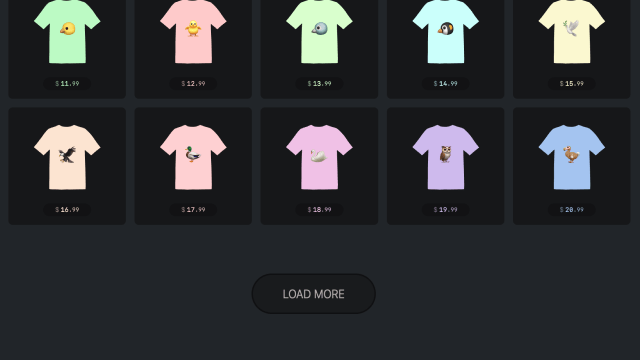
Infinite Scroll - 1/2
Load more items as you scroll down the page.
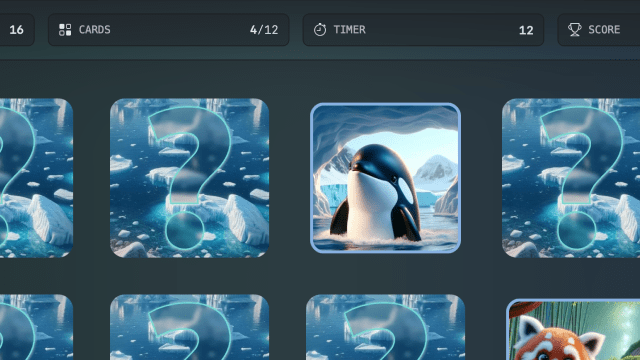
Live Memory Card Game
A Memorable Game UX with Live Components!
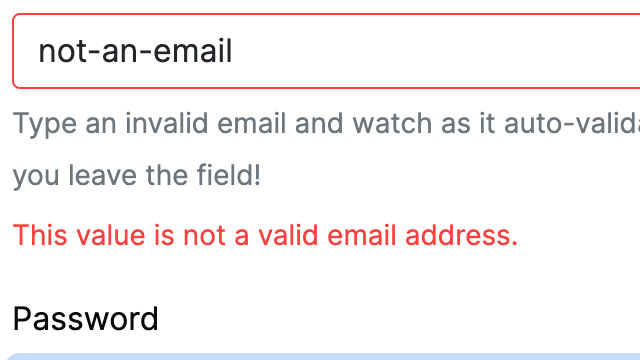
Auto-Validating Form
Create a form that validates each field in-real-time as the user enters data!
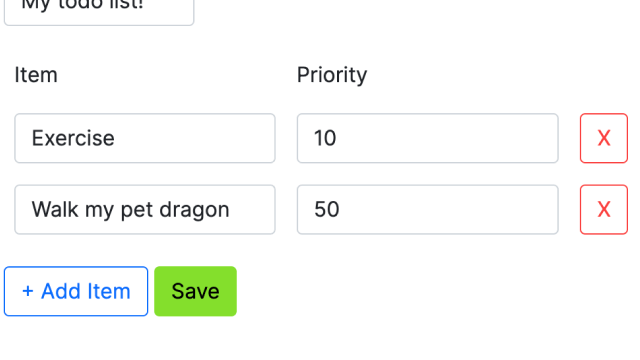
Embedded CollectionType Form
Create embedded forms with functional "add" and "remove" buttons all in Twig.
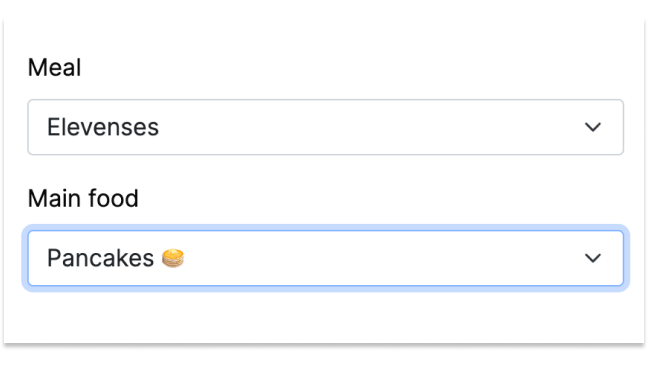
Dependent Form Fields
After selecting the first field, automatically reload the options for a second field.
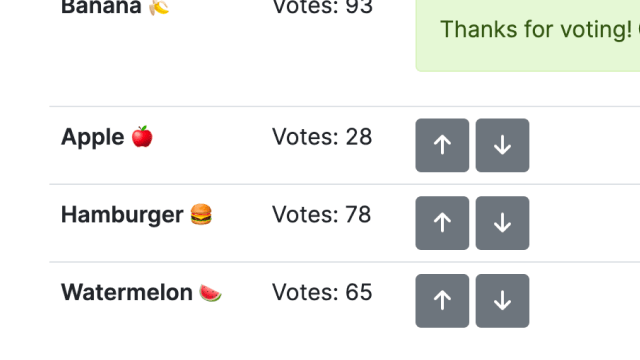
Up & Down Voting
Save up & down votes live in pure Twig & PHP.
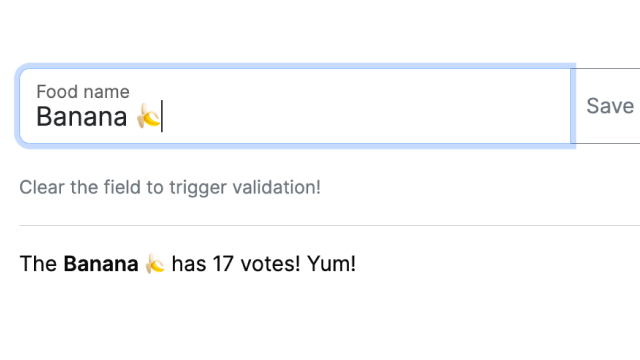
Inline Editing
Activate an inline editing form with real-time validation.
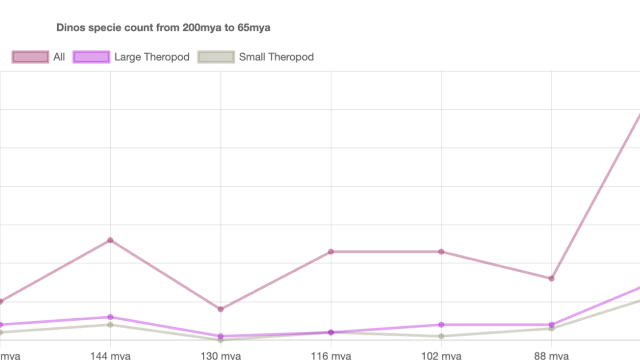
Auto-Updating Chart
Render & Update a Chart.js chart in real-time.
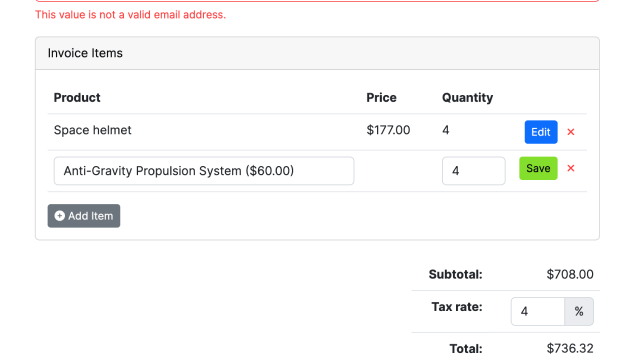
Invoice Creator
Create an invoice + line items that updates as you type.
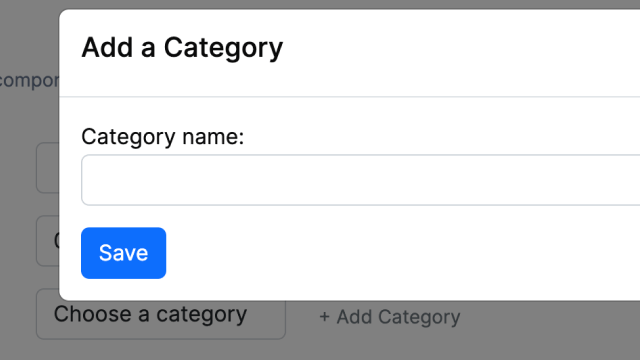
Product Form + Category Modal
Create a Category on the fly - from inside a product form - via a modal.
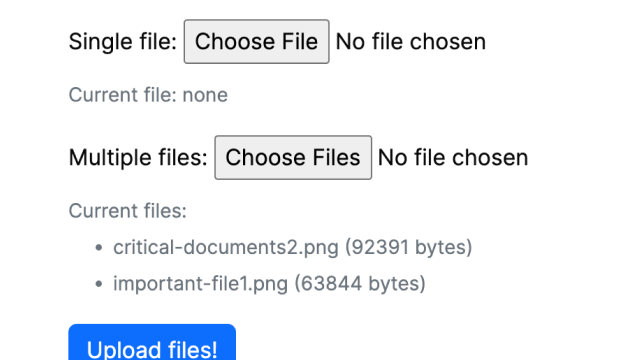
Uploading files
Upload file from your live component through a LiveAction.